Back to posts.
Hair simulation
A while ago I implemented the hair simulation algorithm which is described
in the paper Fast Simulation of Inextensible Hair and Fur by M.Müller,
T.Y.Kim and N.Chentanez, using position based simulation. The simulation
runs in real time can and handle quite some hairs (on CPU).
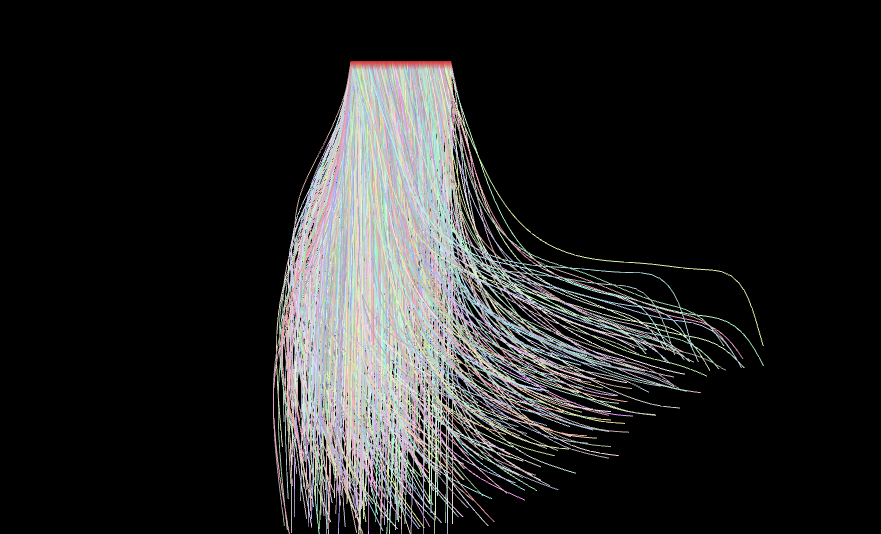
FTL.cpp
#include <glr/GL.h>
#include "FTL.h"
using namespace gl;
namespace ftl {
Particle::Particle(Vec3 p, float m)
:position(p)
,tmp_position(p)
,enabled(true)
{
if(m < 0.001) {
m = 0.001;
}
mass = m;
inv_mass = 1.0 / mass;
}
// ------------------------
FTL::FTL()
:len(10)
{
}
void FTL::setup(int num, float d) {
float dim = 50;
len = d;
Vec3 pos(300, 300, 0);
float mass = rx_random(1.0f, 10.0f);
for(int i = 0; i < num; ++i) {
Particle* p = new Particle(pos, mass); //rx_random(1.0f, 10.0f));
particles.push_back(p);
pos.y += d;
}
particles[0]->enabled = false;
}
void FTL::addForce(Vec3 f) {
for(std::vector<Particle*>::iterator it = particles.begin(); it != particles.end(); ++it) {
Particle* p = *it;
if(p->enabled) {
p->forces += f;
}
}
}
void FTL::update() {
float dt = 1.0f/20.0f;
// update velocities
for(std::vector<Particle*>::iterator it = particles.begin(); it != particles.end(); ++it) {
Particle* p = *it;
if(!p->enabled) {
p->tmp_position = p->position;
continue;
}
p->velocity = p->velocity + dt * (p->forces * p->inv_mass);
p->tmp_position += (p->velocity * dt);
p->forces = 0;
p->velocity *= 0.99;
}
// solve constraints
Vec3 dir;
Vec3 curr_pos;
for(size_t i = 1; i < particles.size(); ++i) {
Particle* pa = particles[i - 1];
Particle* pb = particles[i];
curr_pos = pb->tmp_position;
dir = pb->tmp_position - pa->tmp_position;
dir.normalize();
pb->tmp_position = pa->tmp_position + dir * len;
pb->d = curr_pos - pb->tmp_position; // - curr_pos;
}
for(size_t i = 1; i < particles.size(); ++i) {
Particle* pa = particles[i-1];
Particle* pb = particles[i];
if(!pa->enabled) {
continue;
}
pa->velocity = ((pa->tmp_position - pa->position) / dt) + 0.9 * (pb->d / dt);
pa->position = pa->tmp_position;
}
Particle* last = particles.back();
last->position = last->tmp_position;
}
void FTL::draw() {
glLineWidth(0.1f);
glr_begin(GL_LINE_STRIP);
for(std::vector<Particle*>::iterator it = particles.begin(); it != particles.end(); ++it) {
Particle* p = *it;
if(!p->enabled) {
glr_color(217, 41, 41);
}
else {
glr_color(251, 251, 251);
}
glr_vertex(p->position);
}
glr_end();
}
}
FTL.h
#ifndef ROXLU_FTL_H
#define ROXLU_FTL_H
#include <roxlu/Roxlu.h>
namespace ftl {
struct Particle {
Particle(Vec3 position, float m);
Vec3 position;
Vec3 tmp_position;
Vec3 forces;
Vec3 velocity;
Vec3 d;
float mass;
float inv_mass;
bool enabled;
};
class FTL {
public:
FTL();
void setup(int num, float d);
void addForce(Vec3 f);
void update();
void draw();
public:
float len;
std::vector<Particle*> particles;
Vec3 color;
};
}
#endif
-
NAT Types
This is so exciting, in this article I dive into some of the different ways a NAT device translates addresses which is important for peer-to-peer connections.
-
Building Cabinets
In this post I dive into the design and construction of a cabinet with an interact LED strip. I also explain how I dynamically change the colors of the LEDs over TCP/UDP.
-
Compiling GStreamer from source on Windows
How to compile GStreamer on Windows from Source using Visual Studio 2019 and the meson build system.
-
Debugging CMake Issues
In this post I explain a process you can follow to debug issues with CMake by focusing on a specific target and making the output verbose.
-
Dual Boot Arch Linux and Windows 10
How to install Arch Linux and Windows 10 Pro as dual boot. A step by step tutorial how to create bootable installers, partition and setup a dual boot menu.
-
Mindset Updated Edition, Carol S. Dweck (Book Notes)
Paragraphs I marked from the book "Mindset" from Carol S. Dweck.
-
How to setup a self-hosted Unifi NVR with Arch Linux
A step by step HOW-TO that explain show to setup a Unifi Video Controller with an NFS share with Arch Linux.
-
Blender 2.8 How to use Transparent Textures
Follow this node setup when you want to use an image with transparency as a "sticker".
-
Compiling FFmpeg with X264 on Windows 10 using MSVC
A couple of steps to compile FFmpeg on Windows using MSVC.
-
Blender 2.8 OpenGL Buffer Exporter
The following Blender script creates a [name].h and [name].cpp for the selected object and stores the positions, normals and UVs.
-
Blender 2.8 Baking lightmaps
Light maps are a cheap way to add a lot of realism to you static scenes and have been used forever.
-
Blender 2.8 Tips and Tricks
Use Environment Map only for reflections; create a floor plane for a Product Render, diffuse texture for roughness and more!
-
Setting up a Bluetooth Headset on Arch Linux
Learn how to setup a Sennheiser PXC 550 Bluetooth headset on Arch Linux.
-
Compiling x264 on Windows with MSVC
Compile the excellent x264 source on Windows using MSYS2 and MSVC.
-
C/C++ Snippets
Is a number divisible by four?
-
Reading Chunks from a Buffer
Some thoughts on reading bytes from a file; handy for reading NALs.
-
Handy Bash Commands
Bash scripts: removing white space, lowercase filenames, backup using tar, etc.
-
Building a zero copy parser
Simple solution to parse data in a pretty performant way. Used this for a RTSP protocol parser.
-
Kalman Filter
A very simple yet powerful filter which works great when you have to smooth noisy data. Used for the Nike Rise 2.0 project.
-
Saving pixel data using libpng
Do you have raw RGBA data that you want to save? Use this snippet to save it into a PNG file.
-
Compile Apache, PHP and MySQL on Mac 10.10
Setup you own PHP, MySQL and Apache and with virtual document roots.
-
Fast Pixel Transfers with Pixel Buffer Objects
Using Pixel Buffer Objects (PBO) for fast asynchronous data transfers and OpenGL.
-
High Resolution Timer function in C/C++
Wait...... wait.. fast high resolution timer funtions (Windows, Linux, Mac)
-
Rendering text with Pango, Cairo and Freetype
My never ending obsession with font rendering. A complex beast to do well. Use Pango and FreeType for the heavy lifting.
-
Fast OpenGL blur shader
Make things look blurry ... and fast using this OpenGL blur shader.
-
Spherical Environment Mapping with OpenGL
An old trick to get great lighting effects using Environment Maps and OpenGL.
-
Using OpenSSL with memory BIOs
OpenSSL is a great library with lots of abstractions. In this post I discuss how to break some of these abstractions and use your own memory buffers.
-
Attributeless Vertex Shader with OpenGL
A simple way to render a fullscreen quad without a vertex buffer with OpenGL.
-
Circular Image Selector
Some thoughts on a different way to select images from a huge collection in a compact UI.
-
Decoding H264 and YUV420P playback
Using libav to demux and playback with OpenGL.
-
Fast Fourier Transform
Analyse your audio using the Fastest Fourier Transform in the West.
-
OpenGL Rim Shader
Pretty glowy edges using a GLSL rim shader.
-
Rendering The Depth Buffer
Render the non-linear OpenGL Depth Buffer.
-
Delaunay Triangulation
Do you need to triangulate some shape: use the “Triangle” library.
-
RapidXML
RapidXML is a versatile and fast XML parser with a simple API. Check out these examples.
-
Git Snippets
Some simple GIT snippets; added here to remind myself.
-
Basic Shading With OpenGL
A couple of basic GLSL shaders with explanation.
-
Open Source Libraries For Creative Coding
Collection of great open source libraries for you creative programming projects.
-
Bouncing particle effect
Snippet that can be used to create a bouncy particle effect; basic, effective, simple but nice.
-
OpenGL Instanced Rendering
Want to render thousands and thousands of objects? Use OpenGL instanced rendering. The solution...the only solution.
-
Mapping a texture on a disc
Ever heard about projective interpolation related to texture mapping? Learn about this intertesting issue with OpenGL and texture mapping.
-
Download HTML page using CURL
When you want a quick solution to perform a HTTP(S) request CURL is always a quick an simple solution. Check out this example code.
-
Height Field Simulation on GPU
Although not a Navier-Stokes implementation ... still a very nice and enjoyable effect.
-
OpenCV
Optical Flow: when doing anything with tracking you've probably heard of it. See this simple example code using OpenCV and OpenGL.
-
Some notes on OpenGL
FBOs and Depth Testing, using different Attachment Points, a YUV420p shader, ...
-
Math
Meaning of the Dot Product in 3D graphics, calculating a perpendicular vector using Sam Hocevar's solution, orientation matrix and more.
-
Gists to remember
Some gists that I want to remember, often use, etc...
-
Reverse SSH
Do you want to login, into a remote PC but the remote PC is behind a firewall? Then use this simple reverse SSH trick which doesn't require changing your firewall rules.
-
Working Set
Having issues with your compiler? Or during linking? Check these common issues and their solutions. I also list several tools that you can use to get a some useful info.
-
Consumer + Producer model with libuv
Example of a common Multi Threaded Consumer/Producer Model using LibUV.
-
Parsing binary data
Learn about the basic of a binary protocol and how to create one easily yourself.
-
C++ file operation snippets
Reading a file into a string, vector, checking the file size, change to a position, etc. A collection of C++ file operation snippets.
-
Importance of blur with image gradients
Do you want to experiment with OpenGL and aligning Brush Strokes along Image Gradients? Then check out this post about the importance of blurring.
-
Real-time oil painting with openGL
Code snippet for fake "oil painting" effect with OpenGL using instanced rendering.
-
x264 encoder
Basic example on how to use libx264 to encode image data using libav
-
Generative helix with openGL
Screenshots of a project I worked on with that generates a DNA helix.
-
Mini test with vector field
Screenshots while experimenting with a vector field; nothing much to see here.
-
Protractor gesture recognizer
Testing the amazing One Dollar $1 gesture recognizer. The simplest and very good gesture recognizer.
-
Hair simulation
Example code that implements the "Fast Simulation of Inextensible Hair and Fur" paper from M. Müller, T.Y. Kim and N.Chentanez.
-
Some glitch screenshots
Glitch screenshots.
-
Working on video installation
Screenshots of some experiments of a video installation.
-
Generative meshes
I enjoy creating physics based simulations and render them on high res. Here are some experiments I did a time ago.
-
Converting video/audio using avconv
Examples that show you how to use avconv to manipulate video and audio files.
-
Auto start terminal app on mac
Automatically start you application whe Mac boots and make sure that it restarts your app when it exists. Handy for interactive installations.
-
Export blender object to simple file format
Export the selected object in Blender into a .h and .cpp file that prepresents the buffer.